 |
darkspot Apprentice
Joined: 29 Jul 2002 Posts: 105
|
Posted: Tue Feb 15, 2005 12:21 am
get/put .txt logging. |
I'm trying to make a script that keeps track of how much stuff is given/taken in a given time frame. I can deal with the tiem frame, I'm having trouble keeping track of what is given/taken.
If someone gives then takes something, I want it to cancel itself out
(%w) gets (*) from Shop Vault.
(%w) puts (*) in Shop Vault.
Joe gets a demonskin potion from Shop Vault.
Joe put a demonskin potion in Shop Vault.
it can also show up as another way
(%w) gets (*) ~(%n~) from Shop Vault.
(%w) puts (*) ~(%n~) in Shop Vault.
Joe gets a heal potion (5) from The Container.
Joe puts a heal potion (5) in The Container.
A) I would like to be able to differentiate the two scripts, so that if someone did get or take multiple items I could count that.
B) I was thinking this would be cool to log to a text file.
Basically what I want... if Given the following input
Joe gets a heal potion from Shop Vault.
Joe gets a heal potion (5) from Shop Vault.
Joe gets a heal potion (8) from Shop Vault.
Joe puts a heal potion (5) in Shop Vault.
Joe puts a dragonskin potion (5) in Shop Vault.
Joe gets a shield from Shop Vault.
Joe puts a shield in Shop Vault.
Joe puts a sword in Shop Vault.
I would then have a text file with whatever name (preferably the date)
Of the following content (how it's presented I don't really care, I'd just like it to be generally this)
Joe gets a heal potion from Shop Vault.
Joe gets a heal potion (8) from Shop Vault.
Joe puts a dragonskin potion (5) in Shop Vault.
Joe gets a shield from Shop Vault.
Joe puts a shield in Shop Vault.
Joe puts a sword in Shop Vault.
(Note: the get 5 put 5 canceled each other out)
OR one step futher (which would rock)
Joe gets a heal potion (9) from Shop Vault.
Joe puts a dragonskin potion (5) in Shop Vault.
Joe puts a sword in The Container.
let me know what you can do to help... I'm kinda confused. I was looking into read/write/etc but it looks like it'd be harder to have things cancel themselves out.
I also was just thinking, maybe use a %replace or a #sub somehow...
#sub {(*) (%w) (%w) Shop Vault.} {%1 %2 (1) %3 Shop Vault}
(2 %w's because the second would be either in/from and the first would have to be part of a name of something, aka not (#)) and then make sure the sub hits before the rest of the trigger? just an idea I just thought of ti facilitate.
any questions at all.. let me know, I'll get any other info you need. and if I come up with anything instead I'll post it
----
It doesn't have to log to text... just a preference... if there's another way to keep track of this... that'd be awesome. thing is, I don't want thousands of variables.. and I figured if I used a text file for each instance I start logging this, it would be easier. |
|
|
 |
jptaylor Newbie
Joined: 14 Feb 2005 Posts: 6
|
Posted: Tue Feb 15, 2005 5:15 am |
Hmm. How about instead of a text file use the zMUD database.
The you could add delete entries at will and even group them by who took them or what items.
I haven't had much experiance with the database though,
But I know it's much more versitile then a log file and easier modify. |
|
|
 |
Full Throttle Wanderer
Joined: 07 Dec 2004 Posts: 65
|
Posted: Tue Feb 15, 2005 12:47 pm |
Try this-
Code: |
#class Stock_List
#var stockdb 0 0
#alias stock {#loopdb @stockdb {#show {%key (%val)}}}
#trigger {^(%w) gets (*) from Shop Vault.} {
#if (@stockdb = 0) {#var stockdb %null}
#var getskey %subregex("%1: %2"," \(\d+\)")
#if (%regex(%2,"(\d+)",getsval) = 0) {#var getsval 1}
#if %iskey(@stockdb,@getskey) {
#addkey stockdb {@getskey} {%eval(%db(@stockdb,@getskey)+@getsval)}} {
#addkey stockdb {@getskey} {@getsval}}
#loopdb @stockdb {#if (%val = 0) {#delkey stockdb %key}}
#unv getskey
#unv getsval}
#trigger {^(%w) puts (*) in Shop Vault.} {
#if (@stockdb = 0) {#var stockdb %null}
#var putskey %subregex("%1: %2"," \(\d+\)")
#if (%regex(%2,"(\d+)",putsval) = 0) {#var putsval 1}
#if %iskey(@stockdb,@putskey) {
#addkey stockdb {@putskey} {%eval(%db(@stockdb,@putskey)-@putsval)}} {
#addkey stockdb {@putskey} {-@putsval}}
#loopdb @stockdb {#if (%val = 0) {#delkey stockdb %key}}
#unv putskey
#unv putsval}
#class 0 |
Example Input-
#echo Joe gets a heal potion from Shop Vault.
#echo Joe gets a heal potion (5) from Shop Vault.
#echo Joe gets a heal potion (8) from Shop Vault.
#echo Joe puts a heal potion (5) in Shop Vault.
#echo Joe puts a dragonskin potion (5) in Shop Vault.
#echo Joe gets a shield from Shop Vault.
#echo Joe puts a shield in Shop Vault.
#echo Joe puts a sword in Shop Vault.
stock
Example Output-
Joe: a heal potion (9)
Joe: a dragonskin potion (-5)
Joe: a sword (-1) |
|
|
 |
Full Throttle Wanderer
Joined: 07 Dec 2004 Posts: 65
|
Posted: Tue Feb 15, 2005 2:09 pm |
This is simpler, and works for different shops-
Code: |
#class Stock_List
#var stockdb 0 0
#alias stock {#loopdb @stockdb {#show {%key (%val)}}}
#alias stock0 {#var stockdb 0}
#trigger {^(%w) ({gets|puts}) (*) {from|in} (*).} {
#if (@stockdb = 0) {#var stockdb %null}
#var stockkey %subregex("%4, %1: %3"," \(\d+\)")
#if (%regex(%3,"(\d+)",stockval) = 0) {#var stockval 1}
#math stockval %if(%2 = "puts","-1","1")*@stockval
#if %iskey(@stockdb,@stockkey) {
#addkey stockdb {@stockkey} {%eval(%db(@stockdb,@stockkey)+@stockval)}} {
#addkey stockdb {@stockkey} {@stockval}}
#loopdb @stockdb {#if (%val = 0) {#delkey stockdb %key}}
#unv stockkey
#unv stockval}
#class 0 |
Example Output-
Shop Vault, Joe: a heal potion (9)
Shop Vault, Joe: a dragonskin potion (-5)
Shop Vault, Joe: a sword (-1) |
|
|
 |
darkspot Apprentice
Joined: 29 Jul 2002 Posts: 105
|
Posted: Thu Feb 17, 2005 5:26 am |
that seems to work. Is there anyways of converting databases to simpler forms of file? My friend doesn't use zmud, but we share the shop (I've tried to convert... he's too cheap) but he'd like a copy of the records, and I have no clue if there is a way to do that?
there some forall? like maybe at the end of each time period.. is there some way...
#forall {each database entry} {#write (entry)}
#clear database
I've never been able to work databases that well yet, I've stuck to string lists for a long time, but It keeps getting harder and harder to do so.
Btw.. thanks a MILLION for the help. |
|
|
 |
nexela Wizard
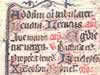
Joined: 15 Jan 2002 Posts: 1644 Location: USA
|
Posted: Thu Feb 17, 2005 5:42 am |
Here is a lil rewrite of an alias I use to log my Database Records. (The file will be in your zmud.exe directory)
#ALIAS writetofile {
#FILE 5 stockDB.txt
#WRITE 5 {%time( c)}
#WRITE 5 {%expanddb( @pathfind, %crlf, ": ")}
#WRITE 5 {%cr}
#WRITE 5 {---------------}
#WRITE 5 {%cr}
#CLOSE 5
} |
|
|
 |
Full Throttle Wanderer
Joined: 07 Dec 2004 Posts: 65
|
Posted: Thu Feb 17, 2005 11:44 am |
This script can store the stockdb database variable between sessions.
Code: |
#class Stock_List
#alias stocksave {#file 1 stockINI.txt
#erase 1
#file 1 stockINI.txt
#write 1 {~#variable stockdb ~"@stockdb~"}
#close 1}
#alias stockload {#read stockINI.txt}
#class 0 |
|
|
|
 |
|
|