 |
daarklord Beginner
Joined: 22 Mar 2011 Posts: 12 Location: nilgiri.mythril.com
|
Posted: Thu Mar 24, 2011 3:21 pm
[resolved] scripting conveniences |
Three things.
One:
Is there a shortcut to increment a variable?
Code: |
#PRINT "begin"
$count = 0
#WHILE ($count<10) {
#PRINT "step"
$count++ //++ is my friend
#WAIT 100
}
#PRINT "end" |
Did not work.
Two:
I wanted to end the infinite loop that occurred from the above code, but I am not aware of any shortcut to end script processes, and I ended up having to close session, and log back on. It would be convenient to have a way to stop the script from running without actually exiting the MUD.
Three:
How do I concatenate strings?
Code: |
#SEND "drink" + $drinkType |
works?
Or what about if I want to add variables into the printed string that I can define later, like in C++ printf style?
Code: |
#SEND "give %d coins to %w" 100 Bob |
Thanks. |
|
Last edited by daarklord on Thu Mar 31, 2011 7:59 am; edited 1 time in total |
|
|
 |
chamenas Wizard

Joined: 26 Mar 2008 Posts: 1547
|
Posted: Thu Mar 24, 2011 3:33 pm |
The best you can hope for is #ADD var num
ex: #ADD $count 1
or
#ADD count 1
I heard that this might be deprecated, but it's still functional either way.
I too have wanted a ++ and -- syntax, but I think the idea was refused when I brought it up.
If there's a condition for breaking the loop you can run a #BREAK in it. If you just mean it's an infinite loop because your increment wasn't working, that stuff happens and infinite loops are nasty things. |
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Thu Mar 24, 2011 4:00 pm |
For your third question, there are two methods of concatenating strings in Cmud--implicit and explicit.
Implicit concatenation is simply putting the strings next to each other:
Code: |
#SEND "drink" $drinkType
#SEND drink $drinktype
#SEND This is method$number
|
Implicit concatenation works much of the time, but there are some cases where it does not work. It is better to use explicit concatenation to avoid potential problems, but implicit concatenation is very common. Explicit concatenation uses a specific function, %concat(), to join strings together:
Code: |
#SEND %concat("drink ", $drinktype)
#SEND %concat("This is method", $number)
|
You also ask about formatted output, which is yet another thing. That can be done with the %format() function. It works much like in C. It allows you to specify the length of insert values as well:
Code: |
#SEND %format("give &d coins to &s", 100, Bob)
#SEND %format("Total value: &4d", $number)
|
|
|
|
 |
chamenas Wizard

Joined: 26 Mar 2008 Posts: 1547
|
Posted: Thu Mar 24, 2011 4:26 pm |
I missed the third question! You can always create your own function too, as fits your needs. 
|
|
|
 |
Anaristos Sorcerer
Joined: 17 Jul 2007 Posts: 821 Location: California
|
Posted: Fri Mar 25, 2011 12:01 am |
While cMUD supports the zMUD legacy, there are other ways to both increment counters and concatenate strings. i.e:
Code: |
$counter = $counter + 1 // equivalent to $counter++
$counter = $counter - 1 // equivalent to $counter--
;;
// There is no compact way to express --$counter or ++$counter in zScript.
;;
$counter = $counter + nn // equivalent to #ADD $counter nn {nn: -maxval < nn < +maxval}
;;
$string = $string1 + string2 // equivalent to %concat( $string1, string2)
$string = $string1 + ... + $stringN // equivalent to %concat( $string1, ...., $stringN)
;;
// Note also that $string1$string2...$stringN will work, especially with commands that take strings such as #ECHO and #WINDOW. I am not sure I would recommend this method, though.
;;
$string = ($string1 + string2) // this is equivalent to %eval( %concat ($string1 ,$string2)) though I am not sure this is official.
|
Note that the suggestions in the above replies are perfectly valid also. |
|
_________________ Sic itur ad astra. |
|
|
 |
shalimar GURU
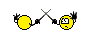
Joined: 04 Aug 2002 Posts: 4686 Location: Pensacola, FL, USA
|
Posted: Fri Mar 25, 2011 12:49 am |
as far as getting out of an infinite loop...
First of you should stick such loops in a separate #THREAD to prevent system lockup, which you can in turn #STOP
#BREAK and #ABORT also have their uses, and sometimes the escape key works as well. |
|
_________________ Discord: Shalimarwildcat |
|
|
 |
chamenas Wizard

Joined: 26 Mar 2008 Posts: 1547
|
Posted: Fri Mar 25, 2011 11:18 am |
I think the string thing was the reason why incrementing operators aren't possible, since zScript variables aren't explicitly typed, and thus it could be attempting to increment a string variable or otherwise, which would be awkward.
|
|
|
 |
daarklord Beginner
Joined: 22 Mar 2011 Posts: 12 Location: nilgiri.mythril.com
|
Posted: Fri Mar 25, 2011 4:52 pm |
Thanks all. I got things working.
edit: For the infinite loops, "#THREAD" then "#STOP <thread ID>" seems to work well.
Now I have another issue, which is not so much of an issue since I can use #ADD to do the same thing but it's a curious thing...
Code: |
//lastActiv is a variable declared earlier
$count = 0
$count = $count + 1 //I can use this kind of expression. Good.
@lastActiv = @lastActiv + 1 //Or can I? |
Doesn't compile. CMUD can add to local variables this way but can't add to non-local variables this way? |
|
|
 |
DraxDrax Apprentice
Joined: 22 Mar 2009 Posts: 149
|
Posted: Fri Mar 25, 2011 5:55 pm |
It doesn't like you using the @ character at the beginning of the line for regular variables.
lastActiv = @lastActiv + 1 //works just fine |
|
|
 |
daarklord Beginner
Joined: 22 Mar 2011 Posts: 12 Location: nilgiri.mythril.com
|
Posted: Fri Mar 25, 2011 6:05 pm |
Yeah I just figured that out. I think @ means you evaluate the variable (like the * in C++), so it's kinda like trying to assign a value to an integer. I don't know what that says about what $ means, but I'm just happy things are working.
|
|
|
 |
oldguy2 Wizard
Joined: 17 Jun 2006 Posts: 1201
|
Posted: Tue Mar 29, 2011 5:31 am |
The $ in front of $count identifies it as a local variable, and yes using the @ is evaluating it. Any time you assign a value to or initialize your variable you don't put a @ in front of it.
|
|
|
 |
|
|