 |
chamenas Wizard

Joined: 26 Mar 2008 Posts: 1547
|
Posted: Mon Jan 19, 2009 9:40 pm
A Couple of Questions about Variables... |
Hey guys, thanks for all of the help you give me. Here's a few more things that should be relatively simple for you to explain, but of which I do not have the answer:
I have seen people try and refer to other variables by the value of another variable, something like @(@var1) where var1="var2", thus the ultimate result of using @var1 in that manner is actually to refer to @var2. My question is wondering if I can use a similar means to create variables that aren't there, and refer to them if they are. And to also see if I can replace any spaces in input with an _.
A few examples:
I want to form an alias with this sort of structure
set [set_type] [equip_slot] [value]
ex:
set mana head helmet blue leather
This alias would first see mana and recognize that as the first value it is a set type (mana gear, hp gear, hit/dam gear, AC gear). It would then see head, and recognize that as the second value is identifying the slot of equipment that I am specifying. In this case, head gear (helmets, hats, etc...). It would then see anything after the first two values as one whole value that might be spaced.
Taking this input, the alias would do the following:
It would create/refer to the appropriate variable, where the variable name is specified by the first two values, in our above example we would be using a variable called mana_head, thus referred to as @mana_head or #var mana_head value
It would then either create the variable if it's not there, or refer to it if it is, and put the last value in as the value of the variable, so ultimately we would have
@mana_head="helmet blue leather"
So, if @mana_head was not there, it would create the variable using value 1 and value 2 and setting the value by value 3. If the variable existed, it would refer to it, using value 1 and value 2 and would replace the current value with value 3.
--------------------------------------------------------
My other question has to do with a similar thing about creating variables.
The following is an affects list of spells currently on a given character.
DSL wrote: |
You are affected by the following spells:
Spell: armor : modifies armor class by -20 for 17 cycles, (8 1/2 hours)
Spell: sanctuary : modifies none by 0 for 2 cycles, (1 hours)
Spell: invisibility : modifies none by 0 for 48 cycles, (24 hours)
Spell: shield : modifies armor class by -20 for 39 cycles, (19 1/2 hours)
Spell: detect invis : modifies none by 0 for 25 cycles, (12 1/2 hours)
Spell: detect hidden : modifies none by 0 for 25 cycles, (12 1/2 hours)
Spell: stone skin : modifies armor class by -40 for 23 cycles, (11 1/2 hours)
|
I have been contemplating creating an elaborate set of variables and triggers to utilize my tick timer and create a separate, status window which constantly gives you and updates affects table showing how many ticks are remaining. For any given spell I need:
Spell: invisibility : modifies none by 0 for 48 cycles, (24 hours)
Spell: ^(\w+)(?:[\w ]+): modifies (?:[\w\-' ]+) by \d+ for (\d+) cycles, \(\d+ (1/2 hours|hours)\)
%1=invisibility
%2=48
I would then use that information gained, via trigger, to create the variable @_invisibility and give it the value 48. If there is already a variable @_invisibility, it would refer to it and replace the current value with 48. So... something like:
#var "_"%1 %2 ??
However, some spells have two words in the name:
Spell: detect invis : modifies none by 0 for 25 cycles, (12 1/2 hours)
Not that difficult to match:
Spell: ^([\w\-' ]+)(?:[\w ]+): modifies (?:[\w\-' ]+) by \d+ for (\d+) cycles, \(\d+ (1/2 hours|hours)\)
%1=detect invis
%2=25
However, when it goes to create the variable it would, if I am correct in my assumptions, create @_detect with the value of "invis 25". That is not what I want. What I do want is: @_detect_invis with a value of 25. So I'm wondering if there's a way to take the spaces in %1 and replace them with _ and then proceed to create or refer to the variable.
Thanks for your assistance. |
|
|
 |
calesta Apprentice
Joined: 07 Dec 2008 Posts: 102 Location: New Hampshire, USA
|
Posted: Mon Jan 19, 2009 10:49 pm |
For the alias this seems to work assuming the set type and equipment slot are only ever going to be one word each:
Code: |
<?xml version="1.0" encoding="ISO-8859-1" ?>
<cmud>
<alias name="set" copy="yes">
<value>#var %1_%2 %-3</value>
</alias>
</cmud>
|
This trigger seems to work for the output you provided:
Code: |
<?xml version="1.0" encoding="ISO-8859-1" ?>
<cmud>
<trigger priority="340" regex="true" copy="yes">
<pattern>^Spell: ([-'\w ]+) : modifies [-'\w ]+ by [-\d]+ for (\d+) cycles, \(\d+ (?:\d+/\d+ )?hours\)</pattern>
<value>#var %subchar(%1, " ", "_") %2</value>
</trigger>
</cmud>
|
|
|
|
 |
Tech GURU
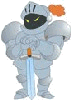
Joined: 18 Oct 2000 Posts: 2733 Location: Atlanta, USA
|
Posted: Mon Jan 19, 2009 10:55 pm |
Well the short answer is yes you can. what you probably need to do is something like this.
Code: |
#var "_"{%subchar(%1," ", "_")} %2 |
or even
Code: |
#var %concat('_',%subchar(%1," ", "_")) %2 |
With that said though I don't think single variables are right data structure for what you want. You will probably be better served by using a single DB variable. Also make sure you set the variable so CMUD doesn't try to store it's data, should make it faster.
However it's completely up to you as to how you code it.
[Edit] I forgot about the subchar piece just substitute piece of it. I just updated it. |
|
_________________ Asati di tempari! |
|
|
 |
chamenas Wizard

Joined: 26 Mar 2008 Posts: 1547
|
Posted: Mon Jan 19, 2009 11:18 pm |
I'll read up on DB variables and see if I'm comfortable enough with them to use them, thanks.
|
|
|
 |
chamenas Wizard

Joined: 26 Mar 2008 Posts: 1547
|
Posted: Tue Jan 20, 2009 1:21 am |
I'm actually failing to see how this will help me, perhaps I should explain more. My idea is not to store information about equipment (though that is a nice feature in the database could be useful for other things). I want to be able to have a way to keep track of sets of gear to be worn and changed in and out of easily.
So I would do something like
Set Mana Head Helmet Blue Leather
Set AC Head Helmet Hard Leather
Set HD head Helmet Spiked Leather
This would create the variables and their values:
@_Mana_Head="Helmet Blue Leather"
@_AC_Head="Helmet Hard Leather"
@_HD_Head="Helmet Spiked Leather"
Then, in a fight, I might type:
wear HD Head
which would send:
get 'Helmet Spiked Leather' container
wear 'Helmet Spiked Leather'
put 'Helmet Blue Leather' container
_Current_Head="Helmet Spiked Leather"
I could also do
wear HD all
which would do the same, except for all equipment slots, etc... |
|
|
 |
gamma_ray Magician
Joined: 17 Apr 2005 Posts: 496
|
Posted: Tue Jan 20, 2009 1:59 am |
I, personally, would probably set it up something like this:
Variables:
A simple string list with all of the slot types in it (head|body|arms)... called @slots.
A variable @armour_current which is a DB variable of all the armour you're wearing right now.
Code: |
body=chain mail|arms=sleeves|head=helmet hard leather |
A db variable for each slot, which lists the different armour types, in the form of @armour_SLOTNAME.
Code: |
mana=helmet blue leather|hd=helmet spiked leather|ac=helmet hard leather |
Aliases:
Set (takes arguments like "set mana head helmet blue leather"):
Code: |
#addkey {armour_%2} {%1} {%-3} |
Wear (takes arguments like "wear mana head" or "wear mana all"):
Code: |
#if (%2 == "all") {
#for @slots {wear %1 %i}
} {
#if (%iskey( @{armour_%2}, %1)) {
get '@{armour_%2.%1}' from container
#send {wear '@{armour_%2.%1}'}
put '@{armour_current.%2}' container
armour_current.%2 = @{armour_%2.%1}
}
} |
And that's everything. I'd be a tiny bit happier if you changed the alias names to something less likely to conflict (you could then get rid of the pesky #send in the wear alias) but other than that, I think everything works. |
|
|
 |
chamenas Wizard

Joined: 26 Mar 2008 Posts: 1547
|
Posted: Tue Jan 20, 2009 2:20 am |
That's a lot of stuff to digest, but I'll definitely try it.
|
|
|
 |
gamma_ray Magician
Joined: 17 Apr 2005 Posts: 496
|
Posted: Tue Jan 20, 2009 11:48 am |
You probably also want to add a quick check to see if you're wearing what you would be wearing when you changed and then do nothing if that's the case.
And a script to grab what you're currently wearing off of something other than having kept track (in case you get something forcibly removed or whatever).
And other stuff I've already forgotten. I hate being old. :/ |
|
|
 |
chamenas Wizard

Joined: 26 Mar 2008 Posts: 1547
|
Posted: Tue Jan 20, 2009 12:54 pm |
As to the second one, if I also stored in the database the short name of the item I could do such a thing. So I suppose it would look like the current with another slot for the short name which is irrelevant until I type EQ and get a list of gear.
The short name can be grabbed if I a devise a way to have it add the short that is given when I wear the particular piece of gear. Something I may have to ask about later, but I just want to get the basics of this whole thing down first. |
|
|
 |
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|