 |
Wyndle Beginner
Joined: 28 Mar 2007 Posts: 20 Location: < here and there >
|
Posted: Sun Apr 01, 2007 4:47 pm
Need help with matching part of an array |
I want to create an array of junk items that I would like to drop automatically upon reciept. Every item has at least two words in the short title (ex. a rusty key, an igloo, the fuzzy black bag, etc.). Before I run this code I want to have someone glance over it for any major errors (like how to match a single entry in the JunkList array). For sake of example, assume the previous examples are the current items populating the array JunkList.
Code: |
#alias addtojunklist {#additem JunkList {%1}}
#alias junkdrop {drop ~'%1~';sacrifice ~'%1~'}
#trigger {^You take %w (*) from the corpse of} {#if (%1 =~ @JunkList) {junkdrop %1} {}} |
Any suggestions or comments are welcome. |
|
|
 |
Fang Xianfu GURU
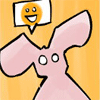
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Sun Apr 01, 2007 6:10 pm |
"=~ @Junklist" may require braces around the variable to work properly. I see %ismember used more frequently than =~ but they're both valid solutions. You also don't need the else braces if there's nothing inside them, though them being there won't hurt. In addition, the apostrophe isn't a special character and probably doesn't need quoting.
One possible problem could come from zMUD's handling of alias parameters. For example:
You take a fuzzy black bag from the corpse of
%1 for the trigger will be "fuzzy black bag" and so "junkdrop fuzzy black bag" is executed. The alias won't see the fuzzy black bag as one parameter even though that's how it was handled by the trigger. The alias will end up sending drop 'fuzzy';sac 'fuzzy' which may not be what you intended. If you intended the alias to send drop 'fuzzy black bag';sac 'fuzzy black bag' then change the %1s in the junkdrop alias to %-1. |
|
|
 |
Wyndle Beginner
Joined: 28 Mar 2007 Posts: 20 Location: < here and there >
|
Posted: Sun Apr 01, 2007 6:41 pm |
So this would be better then?
Code: |
#alias addtojunklist {#additem JunkList {%1}}
#alias junkdrop {drop '%-1';sacrifice '%-1'}
#trigger {^You take %w (*) from the corpse of} {#if (%1 "%ismember @JunkList") {junkdrop %1} {}} |
Or did you mean:
Code: |
{#if (%1 {%ismember @JunkList}) |
I'll leave the else braces so that if I want to later automate adding items to the array I've got a marker for where to put it to remind me.
Edit: after searching for examples of %ismember, perhaps it should be:
Code: |
{#if %1 (%ismember(@JunkList)) |
Edit 2: The working code in use, just in case anyone else wanted to use it:
Code: |
#ALIAS addtojunklist {#ADDITEM JunkList {%1}}
#ALIAS junkdrop {drop %-1;sacrifice %-1}
#TRIGGER {^You take %w (*) from the corpse of} {#IF (%ismember(%1,@JunkList)) {junkdrop %1} {} |
I currently have a whopping total of three items stored in JunkList. I did have to remove the single quotes (' ') from the junkdrop alias since the mud didn't like them. |
|
Last edited by Wyndle on Mon Apr 02, 2007 7:19 pm; edited 2 times in total |
|
|
 |
Fang Xianfu GURU
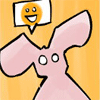
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Sun Apr 01, 2007 8:18 pm |
%ismember(s,l)
returns the location of string s in list l, or 0 if s isn't a member of l. Since #if executes the true command if the expression is greater than 0 and false if the expression is 0 or less, that works out pretty well. %1 is the string you're looking for, and @JunkList is your list, so:
#if (%ismember(%1,@Junklist)) {true} {false}
but like I say, your method is just as valid. The %-1 thing is important, though. |
|
|
 |
Qaiia Wanderer
Joined: 06 Apr 2007 Posts: 59
|
Posted: Mon Jun 30, 2008 4:42 pm |
This little treat should help you out by giving you a right-click context menu to add items to your junk list:
#MENU {Add %selword to junk list} {#ADDITEM JunkList %selword} "" |
|
|
 |
Worgzark Beginner
Joined: 03 Jul 2008 Posts: 10
|
Posted: Fri Jul 11, 2008 12:13 am |
Shouldn't it just be #IF (%ismember(%1,@JunkList)) {junkdrop} {} ? The extra %1 in there seems pointless.
|
|
|
 |
Fang Xianfu GURU
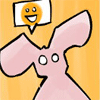
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Fri Jul 11, 2008 12:41 am |
No, because his junkdrop alias needs to know what it is he's going to be dropping. If he just put the code of the junkdrop alias in there, he'd need the %1s in so that the right word is sent to the MUD.
|
|
|
 |
Worgzark Beginner
Joined: 03 Jul 2008 Posts: 10
|
Posted: Fri Jul 11, 2008 4:22 am |
#alias junkdrop {drop '%-1';sacrifice '%-1'} doesn't the %-1 already tell it what it's dropping?
|
|
|
 |
Fang Xianfu GURU
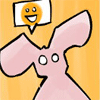
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Fri Jul 11, 2008 9:28 am |
Well, yes, but that's the %-1 for the junkdrop alias and not for the trigger that called it. %1's value within the junkdrop alias is whatever the first parameter of the alias is, even if that means it's blank. It doesn't retain the value from the trigger.
So in order for the value to be passed to the alias properly, it has to be added after the name of the alias. |
|
|
 |
Worgzark Beginner
Joined: 03 Jul 2008 Posts: 10
|
Posted: Fri Jul 11, 2008 5:01 pm |
Ahh ok I thought whatever was stored in %1 by the (*) in his trigger would stay there until there was another value stored in %1.
The problem I had with this kind of trigger was the keywords are not all ways in the same position in the description and the whole description often does not work when performing a mud command. E.g. an old black bag of doom, light of decay and a battle-bow. Drop ‘an old black bag of doom', 'light of decay', 'a battle-bow' does not work. You need to drop 'black bag' or 'light' or 'battle-bow' yet these all exist within different positions in the trigger. How do you assign the keywords to %1 from a trigger when the (%w) position changes? You could have it drop every possible combination but that’s not a practical application.
In the end I assigned keywords to various lists. However this is still not perfect because of similarities between objects. I might want to drop a rusty dagger but not a ruby dagger. I’ve looked at data bases but I can't get my head around how to use them the same way I can use a list with the %ismember function. Could I using a database store rusty dagger and not ruby dagger and have an #IF %ismember type script recognise the difference and drop only the rusty dagger? |
|
|
 |
Fang Xianfu GURU
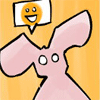
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Fri Jul 11, 2008 8:19 pm |
It's an extremely knotty problem and there's no easy solution, because there's no way for the client to guess what's a keyword and what's not without just trying everything that's not a preposition.
|
|
|
 |
|
|