 |
omniwing Beginner
Joined: 02 Jun 2006 Posts: 19
|
Posted: Tue Jan 01, 2008 9:14 pm
Idle check code concept help |
Hi there,
Here is what I'm trying to accomplish. On a MUD I play, zones idle, and the way you can check to see if a zone is idle is to send a tell to a mob in it. So, for example, if I wanted to see if the chessboard was idle,
tell pawn hello
no-one here by that name. <----- means the zone is idle
you tell a pawn, 'hello' <------ means the zone is not idle
Now, there are about 20 zones that I check very often to see if they are idle. I do this by simply using an alias to send a tell to each of the 20 monsters, and saying their name outloud right after I send them a tell, so if it says 'nobody here by that name' I know which monster /zone is idle.
I want to write code that will have the result of showing me in a new pop up window which zones are idle. The *only* way that I can come up with doing this is:
Make a seperate trigger class for EACH of the 20 monsters. Each class will be defaulted to on. Each class will have a exec that turns all the other classes except itself off, then sends a tell to the specified mob. IF I get 'no-one here by that name', then a variable defined for that mob is set to true, and if I get "you tell (whatever) hello", then the variable is set to false, and the trigger class is turned off.
Then, open up a new window set up to show all true variables from this list.
Now, obviously this is a very roundabout way of doing this, but I can't figure out how to do it more efficently, since 'no-one here by that name' is the response you get from *any* mob that is idle. Can someone help me out with my concept and code? Thank you.
Sorry if this was hard to understand - if you have questions I'll answer them. Thanks again for your time in advance!
-Omni |
|
|
 |
Arminas Wizard
Joined: 11 Jul 2002 Posts: 1265 Location: USA
|
Posted: Tue Jan 01, 2008 10:49 pm |
This code does what you are saying. I may have gotten it exactly backwards but it should be relatively easy to understand once you look at it.
Code: |
#CLASS {ZoneCheck}
#ALIAS cZones {ZoneList="";#loopdb @Zones {#addi ZoneList %key};#T+ zCheckI;#T+ zCheckNI;cZonesA}
#ALIAS cZonesA {#if (@ZoneList != "") {cZone=%pop( ZoneList);tell %item( %db( @Zones, @cZone), 1) hello} {#if (@cZone != "") {#T- zCheckI;#T- zCheckNI;dZoneS}}}
#ALIAS dZoneS {cZone="";#loopdb @zones {#if (%item( %val, 2)==1) {#window IdleZones %key is Idle.}}}
#VAR Zones {zone1(mob1|1)Zone2(mob2|0)Zone3(mob3|1)Zone4(mob4|1)}
#VAR czone {} {_nodef} "ZoneCheck"
#VAR ZoneList {} {_nodef} "ZoneCheck"
#TRIGGER "zCheckI" {no-one here by that name.} {#addkey zones @czone {(%item( %db( @zones, @czone), 1)|1)};cZonesA} "" {disable}
#TRIGGER "zCheckNI" {you tell *, 'hello'} {#addkey zones @czone {(%item( %db( @zones, @czone), 1)|0)};cZonesA} "" {disable}
#CLASS 0
#ALIAS cZones {ZoneList="";#loopdb @Zones {#addi ZoneList %key};#T+ zCheckI;#T+ zCheckNI;cZonesA} "ZoneCheck"
#ALIAS cZonesA {#if (@ZoneList != "") {cZone=%pop( ZoneList);tell %item( %db( @Zones, @cZone), 1) hello} {#if (@cZone != "") {#T- zCheckI;#T- zCheckNI;dZoneS}}} "ZoneCheck"
#ALIAS dZoneS {cZone="";#loopdb @zones {#if (%item( %val, 2)==1) {#window IdleZones %key is Idle.}}} "ZoneCheck"
#VAR Zones {} {_nodef} "ZoneCheck"
#addkey Zones Zone1 {mob1|0}
#addkey Zones Zone2 {mob2|0}
#addkey Zones Zone3 {mob3|0}
#addkey Zones Zone4 {mob4|0}
#VAR czone {} {_nodef} "ZoneCheck"
#VAR ZoneList {} {_nodef} "ZoneCheck"
#TRIGGER "zCheckI" {no-one here by that name.} {#addkey zones @czone {(%item( %db( @zones, @czone), 1)|1)};cZonesA} "ZoneCheck" {disable}
#TRIGGER "zCheckNI" {you tell *, 'hello'} {#addkey zones @czone {(%item( %db( @zones, @czone), 1)|0)};cZonesA} "ZoneCheck" {disable} |
You will need to edit the Zones variable.
Zone1... is the zone name.
mob1... is the name of the mob that you need to speak to.
The Zeros are stating that the zones are NOT idle.
Just enter the zone names and the mob names and when you are ready type cZones.
To install it just highlight the code in the box, copy it into the command line of Zmud all at once and hit enter.
Good luck. |
|
_________________ Arminas, The Invisible horseman
Windows 7 Pro 32 bit
AMD 64 X2 2.51 Dual Core, 2 GB of Ram |
|
|
 |
Arminas Wizard
Joined: 11 Jul 2002 Posts: 1265 Location: USA
|
Posted: Tue Jan 01, 2008 10:53 pm |
Because I didn't want you to have to enter in EVERY mobs name into the trigger I left the matching pattern a * if you wanted you could make it a list or even a list variable.
#var mobs {mob1|mob2|mob3|mob4}
#TRIGGER "zCheckNI" {you tell a {@mobs}, 'hello'} {#addkey zones @czone {(%item( %db( @zones, @czone), 1)|0)};cZonesA} "ZoneCheck" {disable}
Anyway, because of this it would be a good idea if you did not speak to anyone until the script is through running. It should run rather quickly anyway. |
|
_________________ Arminas, The Invisible horseman
Windows 7 Pro 32 bit
AMD 64 X2 2.51 Dual Core, 2 GB of Ram |
|
|
 |
Fang Xianfu GURU
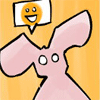
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue Jan 01, 2008 11:06 pm |
Seems like a very long-winded way to do it. Why not just:
#var MobList {pawn|haddock|santa}
#alias CheckZones {#t+ MobList;#forall @MobList {tell %i hello};#var TempMobList @MobList;#alarm +3 {#t- MobList;#say The following mobs did not respond:;#forall @TempMobList {#say %i}}}
#trig "MobList" {You tell ({@MobList}) hello} {#delitem TempMobList %1} "" {disable}
If you wanted to give the name of the zone and not the name of the mob, you could use a dbvar instead like this:
#var MobList ""
#additem MobList pawn "The Chessboard"
#additem MobList haddock "The Fishpond"
#additem MobList santa "Santa's Grotto"
#alias CheckZones {#t+ MobList;#var TempMobList "";#loopdb @MobList {tell %key hello;#additem TempMobList %key};#alarm +3 {#t- MobList;#say "The following zones are idle:";#forall @TempMobList {#say %db(@MobList,%i)}}}
#trig "MobList" {You tell ({@MobList}) hello} {#delitem TempMobList %1} "" {disable} |
|
|
 |
omniwing Beginner
Joined: 02 Jun 2006 Posts: 19
|
Posted: Tue Jan 01, 2008 11:15 pm Thank you |
Working to integrate this now. Thank you for your assistance, I'll post again later on tonight with my results!
-Omni |
|
|
 |
omniwing Beginner
Joined: 02 Jun 2006 Posts: 19
|
Posted: Wed Jan 02, 2008 12:11 am |
Fang,
I'm working on this now, and the problem I'm having is it tells me every mob did not respond, even if they do get sent a tell. Something I noticed, my syntax on my mud is:
you tell santa, 'hello'
instead of
you tell santa hello
So I modified your script thusly:
#var MobList {pawn|haddock|santa}
#alias CheckZones {#t+ MobList;#forall @MobList {tell %i hello};#var TempMobList @MobList;#alarm +3 {#t- MobList;#say The following mobs did not respond:;#forall @TempMobList {#say %i}}}
#trig "MobList" {You tell ({@MobList}), 'hello'} {#delitem TempMobList %1} "" {disable}
That is to say, the only thing I changed was adding comma and single quotes in the trigger in the bottom line, to reflect the proper syntax of my mud. This still doesn't make it work though.
Also, what does #T- do, does this turn off the trigger itself? I thought you could only turn off trigger classes.
Thanks
-Omni |
|
|
 |
omniwing Beginner
Joined: 02 Jun 2006 Posts: 19
|
Posted: Wed Jan 02, 2008 12:55 am |
I tried putting tildes~ infront of each single quote and the comma just incase ZMUD thought they wern't literals, so,
#trig "MobList" {You tell ({@MobList})~, ~'hello~'} {#delitem TempMobList %1} "" {disable}
as the last line, but this didn't work either. Do you think you could explain exactly how this trigger works? It looks to me that there is no removal of the idle mobs when @moblist is changed to @tempmoblist.
Thanks
-Omni |
|
|
 |
Arminas Wizard
Joined: 11 Jul 2002 Posts: 1265 Location: USA
|
Posted: Wed Jan 02, 2008 1:00 am |
You can indeed turn on and off triggers. In fact you can turn on and off aliases as well. #T-
There were many reasons why I went with the long winded approach.
One of them is your current problem. Look at the text you are receiving from the mud. It is probably similar to the following.
You tell santa claus, 'hello'
or as you said.
You tell a pawn, 'hello'
Note the letter a before the word pawn. The trigger that you are using does not take into account that there may be other letters before and or after the word. |
|
_________________ Arminas, The Invisible horseman
Windows 7 Pro 32 bit
AMD 64 X2 2.51 Dual Core, 2 GB of Ram
Last edited by Arminas on Wed Jan 02, 2008 1:07 am; edited 1 time in total |
|
|
 |
Arminas Wizard
Joined: 11 Jul 2002 Posts: 1265 Location: USA
|
Posted: Wed Jan 02, 2008 1:04 am |
The quotes are not your problem.
If the trigger actually fires then mobs are removed from the list. Your problem is that the trigger is never firing because the pattern is not correct.
Have you tried using the trigger tester tab at all?
The () around the {@MobList} capture whatever word matched, if the trigger matched, into %1 and the #deletitem TempMobList %1 would then remove the one that responded from the list. |
|
_________________ Arminas, The Invisible horseman
Windows 7 Pro 32 bit
AMD 64 X2 2.51 Dual Core, 2 GB of Ram |
|
|
 |
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|