 |
Ashur Novice
Joined: 25 Jul 2011 Posts: 39
|
Posted: Thu Sep 01, 2011 2:17 pm
Timer/Gauge Question |
Is it possible to make a gauge representing a timer? In essence, making an hourglass? It seems like I would just need a variable that updates on the system clock every second....
|
|
|
 |
Ashur Novice
Joined: 25 Jul 2011 Posts: 39
|
Posted: Thu Sep 01, 2011 2:23 pm |
Oops! Apologies, the root of my question was actually how to trigger the gauge to start going. So, on <some input> start counting down ... that's what I need help with. 
|
|
|
 |
geniusclown Magician
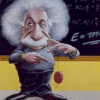
Joined: 23 Apr 2003 Posts: 358 Location: USA
|
Posted: Thu Sep 01, 2011 3:06 pm |
You should use separate triggers: one to set the timer, and another to count down the timer. For example:
Code: |
Timer=0 //create a variable to track the time left
TimerMax=0 //create a variable to use for the max on your gauge
#TR "TimerCountdown" (*1) { //name the trigger "TimerCountdown", and repeat every 1 second
#ADD timer -1 //subtract 1 from time left
#IF (@timer=0) {#T- TimerCountdown} //when the timer gets down to zero, disable the timer so it doesn't go negative
} "" {Alarm} //you can put a class name in the quotes; make the trigger an alarm
#TR {Set timer for (%n) seconds.} { //use whatever pattern you need to start the trigger
Timer=%1 //set the timer to whatever was captured in the pattern
TimerMax=%1 //set the TimerMax variable to use in your gauge.
#T+ TimerCountdown //make sure the alarm trigger is turned on.
} |
I hope this helps! |
|
_________________ .geniusclown |
|
|
 |
Ashur Novice
Joined: 25 Jul 2011 Posts: 39
|
Posted: Thu Sep 01, 2011 3:23 pm |
That is helpful. However, in my case, the TimerMax will always be 60 seconds. I guess the best way to describe the purpose of the timer/gauge is as a cooldown monitor. I know that after I perform a certain action, it will be 60 seconds before I can do it again.
So the possible triggers to start this timer would be something like "You eat a small white berry."
So, then, would I modify the trigger you provided to look something more like this?
Code: |
Timer=0
TimerMax=60
#TR "TimerCountdown" (*1) {
#ADD timer -1
#IF (@timer=0) {#T- TimerCountdown}
}
#TR {You eat a small white berry} {
Timer=60
TimerMax=60
#T+ TimerCountdown
}
|
This might be an obvious question but ... this would be a Trigger correct? I create a trigger with this code in the script text portion? |
|
|
 |
geniusclown Magician
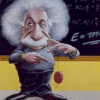
Joined: 23 Apr 2003 Posts: 358 Location: USA
|
Posted: Thu Sep 01, 2011 3:39 pm |
When you see code like this, you can copy it and paste it into the command line, and CMUD will create the appropriate settings. You can then view and change the settings in the Package Editor.
Since your timer will always be one minute, it makes the code even simpler. You won't need a TimerMax variable because it doesn't vary, so just put 60 in the Max field of the gauge and be done with that. So it can be simplified to:
Code: |
Timer=0
#TR "TimerCountdown" (*1) {
#ADD timer -1
#IF (@timer=0) {#T- TimerCountdown}
} "" {Alarm} //This is necessary so the trigger runs as an alarm instead of looking for a pattern
#TR {You eat a small white berry} {
Timer=60
#T+ TimerCountdown
} |
|
|
_________________ .geniusclown |
|
|
 |
Ashur Novice
Joined: 25 Jul 2011 Posts: 39
|
Posted: Thu Sep 01, 2011 3:45 pm |
Excellent! So then the last part is this: There are multiple triggers that should start this timer ... so is there a better way to do it than
Code: |
Timer=0
#TR "TimerCountdown" (*1) {
#ADD timer -1
#IF (@timer=0) {#T- TimerCountdown}
}
#TR {You eat a * berry} {
Timer=60
#T+ TimerCountdown
}
#TR {You eat a * leaf} {
Timer=60
#T+ TimerCountdown
}
#TR {<however many more times is necessary>} {
Timer=60
#T+ TimerCountdown
}
|
edit: Hmmm, also, copy/pasting into the command line did not seem to work. I get an "Error parsing command: illegal character in expression: *" (this is copying the code in your last post ... perhaps I missed something?) |
|
|
 |
geniusclown Magician
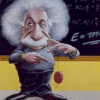
Joined: 23 Apr 2003 Posts: 358 Location: USA
|
Posted: Thu Sep 01, 2011 4:25 pm |
I had improper syntax, my apologies. Use the #ALARM command with braces instead:
Code: |
#ALARM "TimerCountdown" {*1} {
#ADD timer -1
#IF (@timer=0) {#T- TimerCountdown}
} |
Is there a better way? Sure. Assuming eating anything is on the same timer, and the messaging for all eating messages is similar to above, you could do:
Code: |
#TR {^You eat a} {Timer=60;#T+ TimerCountdown} |
Alternatively, if you have a variety of messages, you could create an alias that does the work and just call it:
Code: |
#ALIAS StartTimer {Timer=60;#T+ TimerCountdown}
#TR {^You eat a * berry} {StartTimer}
#TR {^You munch down on some * leaves} {StartTimer}
#TR {^You dine on some * roast} {StartTimer} |
This way, if you decide to make the script more complex (such as keeping track of what you eat or producing messaging in other windows or whatever), then you can just change the single alias and it will affect all of your eating triggers. |
|
_________________ .geniusclown |
|
|
 |
Ashur Novice
Joined: 25 Jul 2011 Posts: 39
|
Posted: Thu Sep 01, 2011 5:31 pm |
I like the alias idea, I'm all about not duplicating code.
I'm still getting the same error when I copy the code into the command prompt....I must be doing something wrong. Is there any prefix that needs added? Am I misunderstanding what the 'command prompt' is altogether?
I feel a bit dumb  |
|
|
 |
geniusclown Magician
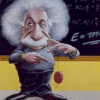
Joined: 23 Apr 2003 Posts: 358 Location: USA
|
Posted: Thu Sep 01, 2011 11:30 pm |
I'm able to copy and paste it in and it did exactly what was expected, so I know the code is good, at least for CMUD v3.34.
Highlight exactly what's in the box (nothing outside of it, nothing excluded), ctrl-c, click in the bar at the bottom of your MUD window where you type to send stuff to the game, ctrl-v, press enter. You should get no message, but will find the setting(s) in your Package Editor.
If you have switched your command input to LUA parsing mode, that would give you errors, too. Pressing ctrl-` (the key above Tab and to the left of 1) will toggle LUA/zScript parsing. To the right of the command line displays a little computer if it's in zScript, and a blue circle icon if it's in LUA. You want the computer. |
|
_________________ .geniusclown |
|
|
 |
Daern Sorcerer
Joined: 15 Apr 2011 Posts: 809
|
Posted: Fri Sep 02, 2011 12:31 am |
Make sure you have parsing on too - if it's off there'll be a red X on the computer in the taskbar that geniusclown mentioned. Just click it or press CTRL+R to turn it back on.
|
|
|
 |
Ashur Novice
Joined: 25 Jul 2011 Posts: 39
|
Posted: Fri Sep 02, 2011 1:16 am |
AH-HAH! It was the parsing thing, for some reason it was turned off.
Okay, the last part (the part that I thought I would be able to do) ... why isn't my gauge working? Here's what I did:
Create new button, change type to gauge, value: @Timer, Max: 60, Low: 0
I've changed the colors as well so I can actually see the bar.
I would expect that the value would update every second, but perhaps this is not so? |
|
|
 |
geniusclown Magician
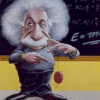
Joined: 23 Apr 2003 Posts: 358 Location: USA
|
Posted: Fri Sep 02, 2011 2:03 am |
Buttons update their displays whenever any (surface level) variable it's displaying changes. So your timer gauge should update whenever the #ALARM fires, each second it's active. Is the @Timer variable positive and the trigger enabled?
By "surface level" variable, I mean regular variables like you're using here, not arrays or indirect references or fancy things like those. |
|
_________________ .geniusclown |
|
|
 |
Ashur Novice
Joined: 25 Jul 2011 Posts: 39
|
Posted: Fri Sep 02, 2011 3:57 am |
As near as I can tell, everything is enabled. If I keep refreshing the variables screen, I can watch Timer count down but the button display always remains the same....empty gauge with white background.
edit: Errr, I just rebooted cmud and it seems to be working now....I didn't change anything, maybe just caused the display to refresh??? |
|
|
 |
|
|