 |
shalimar GURU
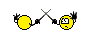
Joined: 04 Aug 2002 Posts: 4734 Location: Pensacola, FL, USA
|
Posted: Fri Dec 30, 2011 6:21 pm
lua database |
Dragging this bit to a new thread, seems appropriate.
charneus wrote: |
Not sure why it's not working for you on that. Hmm.
As for Lua, I've converted many of my scripts (including databases) over to using Lua variables. It would ultimately depend on what you're doing with your script and how you're getting information, but for the #ADDKEY stuff above, it'd look something like:
Code: |
for _,v in pairs(spikeRates) do
v={Failure=0, Success=0}
end
|
Then for the newval part, you could do:
Code: |
newval = spikeRates["steel"]["Failure"]+1
|
Lastly, if you wanted to print the spikeRates database, you could do:
Code: |
for i,v in pairs(spikeRates) do print(i .. ": " .. v) end |
There's a lot to learn about Lua, but it's relatively simple. If you want to get started, you can read through Lua Users Wiki.
Hope this helps you out some. If you want some more help on converting anything over, just let me know and I'll do what I can. By the way, if you want to save those variables for a long run, google table.save lua, save the file in CMUD's Lua directory as tsave.lua, then create an onLoad event in CMUD that does:
Code: |
#LUA {require("tsave")} |
Then to save a table, you just have to execute the command (in Lua): table.save(tablename, "filename you want to save it as") (example: table.save(spikeRates,"spikeRates.tbl")
To recall that table, in the onLoad event, add
Code: |
#LUA {spikeRates=table.load("spikeRates.tbl")} |
It's very useful, and I'm glad Lua is included with CMUD. Just keep in mind that Lua IS case-sensitive. spikeRates is completely different from Spikerates and other variants. |
Remember I am a novice with lua..
Do i just wrap those snippets up into a #LUA command then, like the last example is?
Can i use local variables inside a #LUA command?
I don't see where the first snippet references spike quality either.
I don't see a Lua directory in the CMUD folder as well, I will just go ahead and assume I am to make one. |
|
_________________ Discord: Shalimarwildcat |
|
|
 |
charneus Wizard
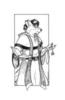
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Fri Dec 30, 2011 7:09 pm |
The Lua directory will be under program files, not the documents folder.
You certainly can wrap them up with the #LUA command, though if you're using extensive Lua, you're better off changing the language to Lua and working from there.
If you use the #LUA command, you can use zscript local variables in it. If you're using strict Lua, you can use Lua's local variables.
I didn't reference the spike quality because I didn't have anything really to reference it. If you provide me with what you currently have (complete) and what you're attempting to do, I can assist you a bit better with that.
I have a quest tracker for Aardwolf that I have nearly all set to Lua, if you want to take a look at it. It might appear jumbled to you, however, as you're still a novice. I may wind up writing up a tutorial and hosting it on my website in the future, though. It's a thought, anyway. |
|
|
 |
shalimar GURU
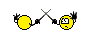
Joined: 04 Aug 2002 Posts: 4734 Location: Pensacola, FL, USA
|
Posted: Fri Dec 30, 2011 8:01 pm |
The variables really take care of themselves at this point, i keep resetting @spikeRates to %null what will the issues i've been having getting the table structure set up.
I have stripped out all the gunk from trying to use zscript alone to do this.
Here is the speedmenu that effectively will run the script
Code: |
<menu priority="12170" id="1">
<caption>spike (highlight the item you are spiking before using)</caption>
<value>$item=%trim( %clip)
#YESNO {You want to spike $item?} {#NOOP} {#EXIT}
$spike=%pick( "p:What kind of spike are you using?", "o:1", "iron", "steel", "silver")
#IF ( @spikeRates.$item) {} {
#SAY {This is your first time spiking $item!}
#IF ( @spikeRates.$item.$spike) {} {
#SAY {This is your first time spiking an $item with a spike of $spike!}
#NOOP Something to set base values and table structure
}
}
itemSpiked=$item
mySpike=$spike</value>
</menu>
|
And then triggers for the pass fail messages returned by the game, i only bothered with one so far since i am trying to iron out the kinks in table structure, referencing.
Code: |
<trigger priority="40" id="4">
<pattern>You ruin the * in the forge!</pattern>
<value>#IF (%null( @itemSpiked) AND %null( @mySpike)) {#EXIT}
$item=%trim( %pop( itemSpiked))
$spike=%trim( %pop( mySpike))
$curValue=@{spikeRates.$item.$spike.Failure}
$newVal=($curValue+1)
#SHOWDB @spikeRates</value>
</trigger>
|
I just hightlight some BS string to test the menu, then use an #echo to make the trigger fire |
|
_________________ Discord: Shalimarwildcat |
|
|
 |
charneus Wizard
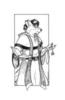
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sat Dec 31, 2011 9:09 pm |
Since most of your menu will need to remain in zscript, we'll change everything from the #IF on, putting them into an event that you can raise in your menu action.
For the event:
Code: |
<?xml version="1.0" encoding="ISO-8859-1" ?>
<cmud>
<event event="onMenuSpike" priority="163290" language="Lua" copy="yes">
<value>itemSpiked, mySpike = zs.param(1), zs.param(2)
if not spikeRates[itemSpiked] then
spikeRates[itemSpiked]={}
print("This is your first time spiking " .. itemSpiked .. "!")
elseif not spikeRates[itemSpiked][mySpike] then
spikeRates[itemSpiked][mySpike]={}
print("This is your first time spiking " .. itemSpiked .. "with " .. mySpike .. "!") -- something to set base values and structure
end
</value>
</event>
</cmud> |
Then, for your menu:
Code: |
<menu priority="12170" id="1">
<caption>spike (highlight the item you are spiking before using)</caption>
<value>$item=%trim( %clip)
#YESNO {You want to spike $item?} {#NOOP} {#EXIT}
$spike=%pick( "p:What kind of spike are you using?", "o:1", "iron", "steel", "silver")
#RAISE onMenuSpike $item $spike</value>
</menu> |
I'm not sure what you're trying to accomplish with your trigger, based on the following observations:
1. You always have itemSpiked and mySpike variables set by your menu. Are you clearing it somewhere that wasn't added here?
2. You set a newVal, but you don't actually change anything in the spikeRates database. Are you needing to add the newVal to the failure?
You also don't need to pop anything since the variables always contain the one element (based on your menu actions). More information will be needed to change your trigger patterns to Lua, too. If you have any questions about the Lua code I provided for the event, let me know.
Edited the Event to allow creation of table entries upon using the Menu action. |
|
Last edited by charneus on Sun Jan 01, 2012 2:58 am; edited 2 times in total |
|
|
 |
shalimar GURU
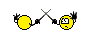
Joined: 04 Aug 2002 Posts: 4734 Location: Pensacola, FL, USA
|
Posted: Sat Dec 31, 2011 11:29 pm |
1. the trigger may go off when someone else attempts a spiking, so I use the variables to error proof and be sure that it is me doing to work (which is why i %pop them)
2. which no proper table structure to be sure i was getting good data, getting the value and setting the new value was not important yet. |
|
_________________ Discord: Shalimarwildcat |
|
|
 |
charneus Wizard
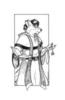
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sun Jan 01, 2012 2:56 am |
Okay, based on my understanding of what you want done, I've composed the code snippet below. I'll provide information to what's going on so you can understand it better, hopefully. I'm also going to modify the information I provided above, so look for an edit in the near future to get the proper update.
Code: |
<?xml version="1.0" encoding="ISO-8859-1" ?>
<cmud>
<trigger priority="40" language="Lua" copy="yes">
<pattern>You ruin the * in the forge!</pattern>
<value>local function addto(t,k,v)
t[k]=t[k]+(v or 1)
end
if itemSpiked and mySpike then
addto(spikeRates[itemSpiked][mySpike],"Failure")
itemSpiked, mySpike = nil, nil
end
for i,v in pairs(spikeRates) do
print(i .. ": " .. v)
end</value>
</trigger>
</cmud> |
The local function part is to make updating table variables slightly easier. The function is a one-script use (much like local variables), so it's not stored into memory afterwards. What it allows you to do is add any value to an existing table key. Without a value, it assumes you're adding 1. In the code above, the addto() function adds 1 to the Failure key of the item.spike table.
Once it's done that, it then erases the itemSpiked and mySpike variables. In Lua, you can assign values to variables with comma-separated entries, like a, b, c, d = 1, 2, 3, 4 will assign 1 to a, 2 to b, etc. Since they are erased, should the trigger fire again without you activating the menu, it'll ignore the commands, but will still show the database.
Speaking of the database, the last snippet of code shows the database. It's equivalent to #LOOPDB where you show the %key and %val. You can modify it even more, if you like, and show only the spiked item's database. Just note that you'll have to move that snippet of code before the values get erased.
The changes I'm going to make to previous code is going to allow for a creation of the table, since in Lua, you'll have to create the table before you can use it. You'll need to initiate things by setting spikeRates to be a table, first and foremost. To do that, just type on the command line:
Code: |
#LUA {spikeRates={}} |
Assuming you have the tsave lua file already, then follow it up with:
Code: |
#LUA {table.save(spikeRates,"spikeRates.tbl")} |
That is, of course, if you're wanting to keep it saved across sessions, since Lua is an "active-memory" only language (meaning that as long as the program runs, Lua will retain values, but as soon as the program quits, all values are lost).
You won't have to define the different elements of the spikeRates table - that'll be done by you using your menu script. Hopefully this helps you get started with Lua and enables you to use it for variable manipulation. I do consider it a bit more manageable for nested databases than CMUD's variables, though they both essentially boil down to json format. |
|
|
 |
shalimar GURU
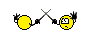
Joined: 04 Aug 2002 Posts: 4734 Location: Pensacola, FL, USA
|
Posted: Sun Jan 01, 2012 1:34 pm |
There is a syntax error on the variable declaration lines of the #EVENT... apparently
syntax checker doesnt seem to llike any of them |
|
_________________ Discord: Shalimarwildcat |
|
|
 |
charneus Wizard
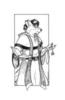
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sun Jan 01, 2012 4:45 pm |
Syntax checker doesn't like to work with Lua. It's designed for zscript only.
Change the variable assignment to two separate lines, then. It should work with that. |
|
|
 |
shalimar GURU
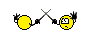
Joined: 04 Aug 2002 Posts: 4734 Location: Pensacola, FL, USA
|
Posted: Sun Jan 01, 2012 5:51 pm |
There has to be something wrong with the #EVENT,
Its still not getting to the point where it will print messages on a dummy entry and neither variable has changed from %null |
|
_________________ Discord: Shalimarwildcat |
|
|
 |
charneus Wizard
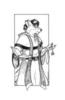
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Mon Jan 02, 2012 12:00 am |
Have you set spikeRates (exactly like that) as a table yet? If you haven't created it, then it definitely won't work.
Are you using the tsave file? Have you set an onLoad event to #LUA {require "tsave"} and to #LUA {spikeRates=table.load("spikeRates.tbl")} (provided you have already saved the database once)? Of course, it'll only load on a fresh opening of CMUD, but you can always force it to load with #RAISE onLoad.
For convenience, I've put the entire tsave contents on pastebin, located here, in case you don't have it yet. I'm assuming, of course, that you want to save the records over time (plus, it will help you in any future endeavors with Lua databases). Again, you'll just need to save it under your lua folder, located in Program Files/CMUD.
In the meantime, I've modified the event to give you something to try. You'll want to delete the if not spikeRates section of code if you implement the tsave, though.
Code: |
<event event="onMenuSpike" priority="163290" language="Lua" copy="yes">
<value>itemSpiked, mySpike = zs.param(1), zs.param(2)
if not spikeRates then
print("You have to set the table first!") -- error message, then sets table
spikeRates={}
end
if not spikeRates[itemSpiked] then
spikeRates[itemSpiked]={}
print("This is your first time spiking " .. itemSpiked .. "!")
elseif not spikeRates[itemSpiked][mySpike] then
spikeRates[itemSpiked][mySpike]={}
print("This is your first time spiking " .. itemSpiked .. " with " .. mySpike .. "!") -- something to set base values and structure
end
</value>
</event> |
Let me know how that works out. |
|
|
 |
shalimar GURU
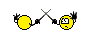
Joined: 04 Aug 2002 Posts: 4734 Location: Pensacola, FL, USA
|
Posted: Mon Jan 02, 2012 2:57 am |
Yes, i did the #LUA command to make it a table,
yes i made the onLoad event (and raised it)
yes i already made the tsave file
The print messages are in fact working now.
the variable definitions still aren't sticking however, not even when i split them up to one per line, they remain %null |
|
_________________ Discord: Shalimarwildcat |
|
|
 |
charneus Wizard
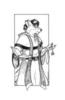
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Mon Jan 02, 2012 3:16 am |
Are you referring to zscript variables remaining %null? They /should/ stick. If the print messages are print out the correct item and spike, then they are being saved.
Keep in mind that none of the above is designed to fill in zscript variables. You can see them at any time by typing #LUA {print(itemSpiked .. " " .. mySpike)} If those are populated (assuming you haven't run the trigger yet), then the trigger should fire properly.
If it's not storing those variables at all, then I'm not sure what the issue can be. By the way, once you get more into Lua, you can toggle the command line to do Lua commands by hitting Ctrl+`. Then you'd just type print(itemSpiked .. " " .. mySpike) instead.
If you're wanting them to be visual in the Settings editor, you can do that, too, by adding these lines:
Code: |
zs.var.itemSpiked = zs.param(1)
zs.var.mySpike = zs.param(2) |
However, I tend not to create extra variables when they aren't really needed. I've managed to stay variable corruption-free for a while now, making a majority of things in Lua. |
|
|
 |
shalimar GURU
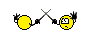
Joined: 04 Aug 2002 Posts: 4734 Location: Pensacola, FL, USA
|
Posted: Mon Jan 02, 2012 1:17 pm |
For some reason i thought this was using the zscript variables still, that was my mistake. I have since deleted all the variables i was using in relation to this.
The only print message that is working is the one that says i've not spiked a given item before.
Or used a given spike.
It never tries to display the table.
I assume this is because the table is never getting keys added to it.
I tried adding a:
print(spikeRates)
to the start of the trigger, just to see what the value was, and it is literally just: [] |
|
_________________ Discord: Shalimarwildcat |
|
|
 |
charneus Wizard
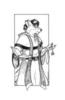
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Fri Jan 06, 2012 1:44 pm |
Sorry I've taken so long to get back to you on this. I've figured out the problem, and I have a working model on my CMUD now. Before I post it here though, I was wanting to get more information from you regarding the script.
1. Are you ever spiking with anything other than iron, steel, or silver?
2. You have a trigger for the failure, so I'm assuming there's one for success. Are you only keeping track of success and failure in the databases per spike? If so, I can have it display like below:
Code: |
Item1
iron : 1 success, 3 failure
steel : 10 success, 4 failure
silver : 3 success, 2 failure
Item2
iron : 3 success, 6 failure
steel : 2 success, 2 failure
silver : 4 success, 3 failure
etc |
If not, give me all that you're keeping track and we will get this working for you. |
|
|
 |
shalimar GURU
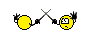
Joined: 04 Aug 2002 Posts: 4734 Location: Pensacola, FL, USA
|
Posted: Fri Jan 06, 2012 3:47 pm |
1. So far, no. But another quality type could potentially get added later.
2. Yes, just a pass/fail tracker.
I was hoping to eventually use this to table to extrapolate out spike rates in four categories:
per item per spike (default view)
per item any spike
any item per spike
any item any spike |
|
_________________ Discord: Shalimarwildcat |
|
|
 |
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|