 |
Decker Newbie
Joined: 18 Dec 2003 Posts: 9
|
Posted: Fri Aug 05, 2005 6:46 am
Database Records and Javascript [SOLVED] |
In a script, I need to do several (above 200) database record operations. I iterate on every key of some database records with #LOOPDB, I change key values with #ADDKEY, etc. However, I found out that ZScript is too slow for my purpose. Thus, I tried to use Javascript and the COM interface to achieve decent performance.
I can read and write plain 'scalar' variables with sess.GetVar(). For database record variables, I have a problem. If I use sess.getVar(), I fetch the whole content of the database. Then, I have to parse the string to fetch the values I'm interested in. Likewise, if I want to update the database, I have to write the whole string:
x = sess.getVar("tmp", "")
x.value = 'a' + String.fromCharCode(30) + 'b' + String.fromCharCode(29) + 'c' + String.fromCharCode(30) + 'd'
What I really need is the following class inheritance:
zMUDObject->zMUDVar->zMUDRecordVar
and the methods getKey(), addKey(), deleteKey() and listKeys().
However, it seems that no such support exists. I can set individual keys with
sess.ProcessCommand('#ADDKEY tmp key value')
and get the value with
x = sess.EvaluateStr('%db(@tmp, key)')
However, since I am using Javascript for performance, I do not want to have zMUD parse commands in ZScript.
Does anyone know the solution to my problem? If there is none (if only getVar() work), I'd be interested in the code that parses the string and puts the values into a hash table (if anyone did that).
Thanks for any help! |
|
Last edited by Decker on Mon Aug 08, 2005 1:49 am; edited 1 time in total |
|
|
 |
Dharkael Enchanter
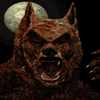
Joined: 05 Mar 2003 Posts: 593 Location: Canada
|
Posted: Sat Aug 06, 2005 4:56 am Re: Database Records and Javascript |
Decker wrote: |
... I'd be interested in the code that parses the string and puts the values into a hash table (if anyone did that).
|
Code: |
var zmud = new ActiveXObject('Zmud.Application');
var db = zmud.CurrentSession.GetVar('testdb','Test');
var hash = {};
var arr =[];
var re = new RegExp("([^\x1E]*)\x1E([^\x1D]*)\x1D?","ig");
while ((arr = re.exec( db.Value )) != null)
hash[arr[1]]=arr[2]; |
In a zmud record variable ascii character 30 (\x1E) separates the key from the value in a key-value pair, ascii character 29 (\x1D) separates one key-value pair from the following key-value pair;The final key-value pair does not have the 29 terminator. |
|
_________________ -Dharkael-
"No matter how subtle the wizard, a knife between the shoulder blades will seriously cramp his style." |
|
|
 |
Decker Newbie
Joined: 18 Dec 2003 Posts: 9
|
Posted: Mon Aug 08, 2005 1:47 am Thanks for the help! |
By your answer, I assume there are no other ways to proceed. Thanks a lot for your code! I finished mine at about the same time as you posted. I'm posting it here in case someone is struck with the same problem.
Code: |
function convertZDB(zdb)
{
var jdb = new Object();
if(zdb != '')
{
var pair_array = zdb.split('\x1D');
for(var i in pair_array)
{
var pair = pair_array[i].split('\x1E');
jdb[pair[0]] = pair[1];
}
}
return jdb;
}
function convertZDB2(zdb)
{
var jdb = new Object();
var re = new RegExp("([^\x1E]*)\x1E([^\x1D]*)\x1D?","ig");
var arr;
while ((arr = re.exec(zdb)) != null)
jdb[arr[1]] = arr[2];
return jdb;
}
function convertJDB(jdb)
{
var zdb = new String();
var first = 1;
for(var key in jdb)
{
if (first) {first = 0;} else {zdb += '\x1D'}
zdb += (key + '\x1E' + jdb[key]);
}
return zdb;
}
function getDB(name)
{
var zdb = sess.getVar(name, '');
return convertZDB(zdb.value);
}
function writeDB(name, jdb)
{
var zdb = sess.getVar(name, '');
zdb.value = convertJDB(jdb);
}
function test()
{
for(var i = 0; i < 1000; i++)
{
var x = getDB('db_to_read');
writeDB('db_to_write', x);
}
}
|
I compared your implementation to mine using the function test(), to see which one is faster. The database I'm reading/writing contains 37 values. It appears my function is slightly faster. Here are the run times of test(), in milliseconds:
My version: 922
Your version: 1250
Your version is more compact, though. Thanks again!
Note to people interested in the code:
Do not forget to create an empty variable for the database you want to read or write. There will be an error if the variable does not exist. |
|
|
 |
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|